Top 18 C# Developers Available Today
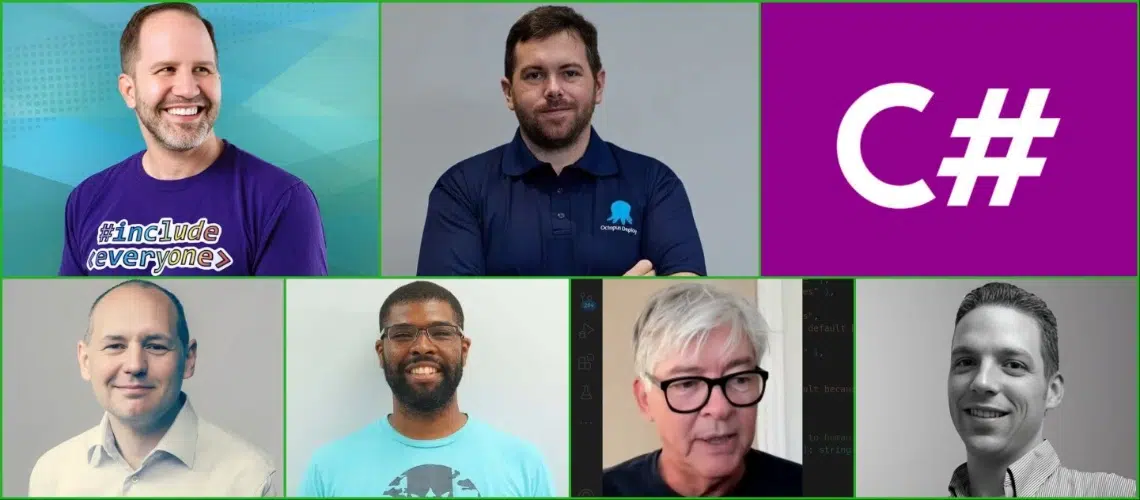
The C# ecosystem thrives thanks to outstanding developers around the world.
Here is a list of the best global CSharp developers (in no particular order), selected for their equally balanced contributions to open-source projects, leadership in tech companies/startups while actively coding, influential blogging/vlogging, impactful roles at major tech firms, and even competitive programming achievements in C#:
- Anders Hejlsberg
- Mads Torgersen
- Scott Hanselman
- Jon Skeet
- Miguel de Icaza
- James Newton-King
- David Fowler
- Oren Eini (Ayende Rahien)
- Konrad Kokosa
- Steve Sanderson
- Stephen Cleary
- Marc Gravell
- Jimmy Bogard
- Nicholas Blumhardt
- Jeremy D. Miller
- Paul Stovell
- Kathleen Dollard
- Julie Lerman
Each of these individuals is actively contributing to the C# community and they come from diverse regions and backgrounds, representing various facets of the developer spectrum. Let’s delve into their profiles and achievements:
Anders Hejlsberg
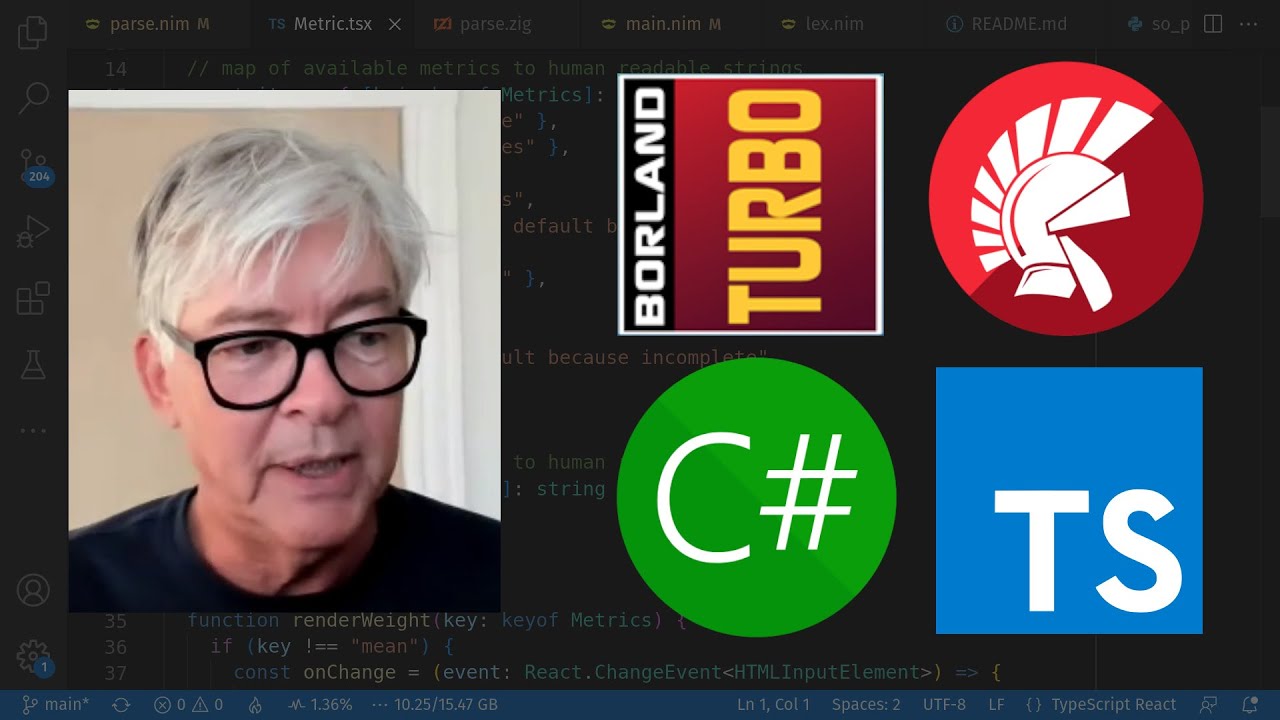
From Turbo Pascal to Delphi to C#
Nationality: Danish
Anders is the original creator of the C# language and a Technical Fellow at Microsoft.
A legendary language engineer, he previously authored Turbo Pascal and was chief architect of Delphi. In recent years, Anders has also been a core developer and lead architect of TypeScript, extending his impact on software development. He remains an active innovator on the C# team, guiding the language’s evolution since its inception in 2000.
Anders’ pioneering contributions and decades of leadership in programming language design make him an undisputed top C# dev of the year.
- LinkedIn: Anders Hejlsberg
- X (Twitter): @ahejlsberg
- GitHub: ahejlsberg
Mads Torgersen
Nationality: Danish
Mads is the Lead Designer of the C# language and an architect on Microsoft’s .NET team.
He has been at the helm of introducing modern C# features and steering the open-source development of the language. Mads is known for his collaborative approach—engaging the community through design meetings and GitHub proposals—to shape each new C# version. Under his guidance, C# has evolved with cutting-edge features (from LINQ to async streams and beyond) while maintaining its hallmark simplicity and power.
An 18-year Microsoft veteran, Mads continues to push CSharp forward in 2025, ensuring it remains a premier language for cloud, mobile, and backend development.
- LinkedIn: Mads Torgersen
- X (Twitter): @MadsTorgersen
- GitHub: MadsTorgersen
Scott Hanselman
Nationality: American
Scott is a prominent C# community figure and Partner Program Manager at Microsoft, celebrated for his outreach and education efforts.
Through his popular blog, podcasts (“Hanselminutes” and “Azure Friday”), and YouTube videos, Scott has spent decades demystifying .NET and inspiring developers worldwide. He actively codes and shares projects on GitHub, often demonstrating the latest C# and .NET techniques in practical scenarios. Hanselman’s influence as a developer advocate is immense – he bridges the gap between Microsoft’s product teams and everyday developers, ensuring technologies like ASP.NET Core, Blazor, and .NET MAUI are approachable.
He continues to be a trusted voice in the CSharp world, leveraging his platform to welcome and mentor the next generation of C# developers.
- LinkedIn: Scott Hanselman
- X (Twitter): @shanselman
- Website/Blog: hanselman.com
Jon Skeet
Code should be written to minimize the time it would take for someone else to understand it.
Nationality: British
Jon is known as the world’s top Stack Overflow contributor, particularly in C#.
He has amassed over 1.5 million reputation points on Stack Overflow – the highest of any user – by answering tens of thousands of C# questions. Jon is also the author of the acclaimed book “C# in Depth”, which has guided many developers through complex language features. A software engineer at Google (working from the UK), he remains deeply connected to the .NET community: he’s a former Microsoft MVP and continues to blog about new C# features and best practices. Jon’s willingness to share knowledge and clarify intricate C# concepts (sometimes even correcting the Microsoft docs or compiler itself) has earned him rock-star status in the community.
His blend of technical expertise and approachability secures his place among the very best.
- X (Twitter): @jonskeet
- GitHub: jskeet
- Website/Blog: codeblog.jonskeet.uk
Miguel de Icaza
Nationality: Mexican
Miguel is a Mexican-American programmer renowned for bringing C# and .NET to non-Windows platforms.
He co-founded the Mono project in 2001, implementing the .NET Framework for Linux, and later co-created Xamarin, which enabled C# development for iOS/Android. Miguel’s work was instrumental in the cross-platform evolution of .NET (Mono now powers Unity3D and Xamarin evolved into .NET MAUI). In 2016, Xamarin was acquired by Microsoft, and Miguel served as a Distinguished Engineer, championing open-source .NET. Though he left Microsoft in 2023, Miguel remains an active open-source advocate and technology innovator (exploring areas like language runtimes and game engines).
His legacy in the CSharp ecosystem – making .NET truly global and open – is profound, and he continues to influence its direction as a respected thought leader.
- LinkedIn: Miguel de Icaza
- X (Twitter): @migueldeicaza
- GitHub: migueldeicaza
- Website/Blog: tirania.org/blog
James Newton-King
Nationality: New Zealander
James is the author of Json.NET (Newtonsoft.Json), the ubiquitous JSON framework that became a de facto standard in .NET development.
Created as a personal open-source project, Json.NET’s reliability and performance led it to be used by millions of developers (and it was even bundled in .NET for many years). In 2019, James joined Microsoft and has since been a driving force on the .NET team, contributing to areas like Azure SDKs and the new System.Text.Json library. His knack for building developer-friendly APIs and tools is well known – his open-source library has over 1 billion downloads on NuGet.
As of today, James continues to build essential .NET infrastructure at Microsoft, and his influence is felt in nearly every C# application that exchanges JSON data.
- LinkedIn: James Newton-King
- X (Twitter): @JamesNK
- GitHub: JamesNK
- Website/Blog: james.newtonking.com
David Fowler
Nationality: Kittitian
David is a Distinguished Engineer at Microsoft and one of the architects behind ASP.NET Core.
Originally from the tiny Caribbean island of Saint Kitts, David co-created the SignalR real-time framework early in his career and quickly became known for his skill in building high-performance networking code. He was a key engineer in the team that rebuilt the .NET web stack (ASP.NET Core and Kestrel) from the ground up to achieve industry-leading performance. David is also very active on GitHub and X (Twitter), where he shares tips on async programming, performance tuning, and new .NET runtime features. Renowned for his “code first” leadership style, he often live-codes prototypes of future .NET features.
David’s fingerprints are on many core .NET developments, and he remains a mentor and inspiration to C# developers aiming to contribute to open source.
- LinkedIn: David Fowler
- X (Twitter): @davidfowl
- GitHub: davidfowl
Oren Eini (Ayende Rahien)
Nationality: Israeli
Oren, also known by his pen name Ayende Rahien, is a prominent Israeli software developer best known for creating RavenDB, a leading NoSQL database for .NET.
A long-time open-source advocate, Oren was a core contributor to NHibernate and other .NET OSS in the 2000s, and eventually founded Hibernating Rhinos, his company, to build RavenDB from scratch. RavenDB (written in C#) pioneered easy ACID transactions in a document database and is used worldwide in production systems. Oren is also a prolific blogger at ayende.com, where he shares deep insights into database internals, performance tuning, and elegant code design. Despite being a CEO, he continues to actively code and push commits to RavenDB’s repository.
Oren’s passion for performance and clean code is reflected in RavenDB’s continued improvements and in his frequent technical posts. He’s considered a “hometown hero” of the European tech scene (often working from Israel and Europe) and remains one of the top C# system developers.
- LinkedIn: Oren Eini
- X (Twitter): @ayende
- GitHub: ayende
- Website/Blog: ayende.com/blog
Konrad Kokosa
Nationality: Polish
Konrad is a Polish .NET performance guru and the author of the definitive book “Pro .NET Memory Management”.
As a Microsoft MVP, Konrad has spent years analyzing and teaching how the .NET garbage collector and memory allocator work, helping developers write more efficient C# code. He co-founded Dotnetos, an organization that runs .NET performance conferences and workshops. Konrad also created tools like Heap Explorer and the Memory Diagnostic Kit to aid profiling. In the community, he’s known as a “Polish .NET legend” and performance expert.
In 2025, Konrad has taken his expertise into new domains as well – he leads an engineering team at a blockchain company (Nethermind) focusing on .NET performance in blockchain tech, showing the versatility of CSharp beyond traditional apps. He remains active in speaking (e.g., at NDC London) and on social media, often sharing low-level .NET tips. Konrad’s influence ensures that performance and memory awareness are top of mind for serious .NET developers.
- LinkedIn: Konrad Kokosa
- X (Twitter): @konradkokosa
- GitHub: kkokosa
Steve Sanderson
Nationality: British
Steve is a developer/architect at Microsoft famous for creative web innovations in .NET.
Based in the UK, Steve originally created Knockout.js (a pioneering MVVM library for JavaScript) and later joined Microsoft’s ASP.NET team. He is the original creator of Blazor, the framework that allows C# to run in the browser via WebAssembly. Steve’s ability to marry C# with cutting-edge web tech has opened new frontiers for .NET developers, from offline PWAs to hybrid server/client web apps. In addition to Blazor, he contributes to ASP.NET Core’s performance and authored popular libraries like WebOptimizer.
Steve is exploring the next generation of Blazor (combining server and WASM models) and remains one of the most inventive minds in the C# world, constantly prototyping features that other frameworks haven’t even dreamt of yet.
- X (Twitter): @stevensanderson
- GitHub: SteveSandersonMS
- Website/Blog: blog.stevensanderson.com
Stephen Cleary
Nationality: American
Stephen is an expert on async/await and concurrency in Csharp, known for his clear and authoritative writing on the subject.
He is the author of “Concurrency in C# Cookbook” and maintains a popular blog where he delves into advanced topics like synchronization contexts, async streams, and cancellation tokens. Stephen’s Stack Overflow contributions and blog posts have made him the go-to person for developers grappling with asynchronous programming pitfalls. He has also created several open-source libraries (such as Nito.AsyncEx for additional synchronization primitives beyond Task) to help the community. As a Microsoft MVP, Stephen frequently speaks at conferences and user groups about best practices in .NET development.
He continues to update his libraries for .NET 8 and regularly publishes deep-dive articles (for example, exploring new async features or improvements in the latest C# versions). Stephen’s dedication to education and high-quality library development cements his status among the top C# developers active today.
- LinkedIn: Stephen Cleary
- X (Twitter): @aSteveCleary
- GitHub: StephenCleary
- Website/Blog: blog.stephencleary.com
Marc Gravell
Nationality: British
Marc is a highly respected engineer who recently joined Microsoft, after over a decade as a principal engineer at Stack Overflow.
He is co-creator of the Stack Exchange network’s architecture, building much of it in C#. Marc co-authored the Dapper micro-ORM (an ultra-fast database library) and the protobuf-net library for efficient serialization. His penchant for writing high-performance C# code has benefited millions of developers — for example, Dapper is a top-used NuGet package. Marc was also a legendary Stack Overflow user (one of the top all-time, especially in C# tag).
Now at Microsoft, Marc works on the .NET team focusing on developer tooling and infrastructure. Known as a “code geek” and CSharp fan, he continues to share insights on Twitter and contribute to open-source (.NET runtime, Roslyn, etc.). Marc’s blend of real-world systems experience and open-source contributions secure his place as one of the top C# developers globally.
- X (Twitter): @marcgravell
- GitHub: mgravell
- Website/Blog: blog.marcgravell.com
Jimmy Bogard
Nationality: American
Jimmy is an independent software architect and a prolific open-source creator in the .NET community.
He created the widely-used AutoMapper library (for object-object mapping) and the MediatR library (implementing the Mediator pattern for CQRS), among other tools. These libraries are staples in enterprise C# applications and have collectively been downloaded millions of times. Jimmy is also known for promoting the “vertical slice architecture” for .NET systems and has shared his knowledge through conference talks, a popular blog, and as an author of the book “ASP.NET Core in Action”. Based in Austin, Texas, he runs a consulting company and actively mentors teams on clean architecture and domain-driven design in C#.
Jimmy’s OSS projects (like AutoMapper) are still very active, and he’s often on X (Twitter) discussing improvements or helping developers – exemplifying a balance of writing code that powers companies and fostering community growth.
- LinkedIn: Jimmy Bogard
- X (Twitter): @jbogard
- GitHub: jbogard
- Website/Blog: jimmybogard.com
Nicholas Blumhardt
Nationality: Australian
Nicholas is the creator of Serilog, the popular structured logging library for .NET, and a founder of Datalust (the company behind Seq, a powerful log analysis tool).
Hailing from Australia, Nicholas has long been an open-source trailblazer in .NET – he also initiated the Autofac dependency injection container back in 2007 (one of the first IoC containers for .NET). As a former program manager at Microsoft (years ago), he helped shape parts of .NET, but his passion clearly lies in building tools that developers love. Serilog introduced the idea of “structured logging” to the .NET world, and its rich ecosystem of sinks and enrichers is a testament to Nicholas’s focus on extensibility.
He maintains Serilog (which recently hit version 3.0 with new features) and actively engages with the community via GitHub and Mastodon. Nicholas’s influence on how C# applications are built (with proper logging and DI practices) is immense and ongoing.
- LinkedIn: Nicholas Blumhardt
- X (Twitter): @nblumhardt
- GitHub: nblumhardt
- Website/Blog: nblumhardt.com
Jeremy D. Miller
Nationality: American
Jeremy is a veteran software architect and the original author of StructureMap, the first widely used dependency injection container for .NET (dating back to 2004).
Jeremy has a passion for architectural patterns and clean code, which he’s demonstrated through his open-source projects over the years. He leads the development of JasperFX libraries – notably Marten (a Postgres-based document database and Event Sourcing library for .NET) and Wolverine (a next-generation background job and messaging framework). These projects push the envelope of what can be done with C# in terms of efficiency and design elegance. Jeremy runs his own company (JasperFX) and consults for enterprise clients, while maintaining an active blog “The Shade Tree Developer” where he shares the lessons learned from real-world systems.
In 2025, Jeremy’s projects Marten and Wolverine are gaining wider adoption in the .NET community for building scalable systems, and he continues to refine them (releasing regular updates and engaging on GitHub). His long-term commitment to improving the .NET ecosystem through open source makes him a standout figure.
- LinkedIn: Jeremy D. Miller
- X (Twitter): @jeremydmiller
- GitHub: jeremydmiller
- Website/Blog: jeremydmiller.com
Paul Stovell
Nationality: Australian
Paul is the founder and CEO of Octopus Deploy, one of the most popular deployment automation tools in the .NET world.
Based in Australia, Paul started Octopus Deploy as a nights-and-weekends C# project in 2011, aiming to simplify the deployment of .NET applications – and it grew into a major DevOps company with hundreds of employees and a $100M+ ARR by 2024. Importantly, Paul never stopped coding; he was the original developer of the Octopus server (written in C# on top of ASP.NET and Azure) and continues to guide its technical evolution. In earlier years, Paul also created open-source libraries like “Bindable LINQ” and contributed to WPF and Silverlight communities.
While Octopus Deploy as a product is mature, Paul remains hands-on in technical decisions and frequently interacts with the community of users via his blog and GitHub. His journey from solo developer to tech CEO exemplifies how far C# can take an entrepreneur, and his active involvement keeps him among the top CSharp practitioners.
- LinkedIn: Paul Stovell
- X (Twitter): @paulstovell
- GitHub: PaulStovell
- Website/Blog: paulstovell.com
Kathleen Dollard
Nationality: American
Kathleen is a Program Manager on Microsoft’s .NET team, overseeing .NET languages (C#/F#/VB) and the developer CLI experience.
With a career spanning decades, Kathleen is also known for her work on code generation and dynamic languages. She wrote “Code Generation in Microsoft .NET” back in 2004 and has been a frequent speaker at conferences like Build and DevIntersection. In recent years, Kathleen has been heavily involved in .NET Core’s open source development – hosting Community Standups, engaging with the community on GitHub for C# and F# language design, and driving improvements to the dotnet CLI and SDK. She was instrumental in pushing Source Generators and analyzers in Roslyn.
Kathleen’s focus is on the developer inner-loop productivity: she’s been advocating for better tooling, templates, and polishing those C# 13 preview features. Her passion for teaching is evident as she loves to talk about code and help developers “fall in love” with new .NET features. As a female leader in the C# world, she also mentors other women in tech. Kathleen’s continued impact on both the language and community makes her one of the top figures in C# today.
- LinkedIn: Kathleen Dollard
- X (Twitter): @KathleenDollard
Julie Lerman
Nationality: American
Julie is a world-renowned authority on Microsoft’s data access tech and a long-time Entity Framework expert.
Based in the U.S., Julie has been a Microsoft MVP for over a decade and is the author of the highly regarded “Programming Entity Framework” book series. She is often called the “leading independent authority on EF”, having guided the community from classic ADO.NET through EF Core in modern cloud apps. Julie is a frequent speaker at international conferences and writes for well-known tech publications (including MSDN Magazine and CODE Magazine) on topics like domain-driven design, EF Core performance tuning, and using C# for data-oriented microservices.
Julie is focusing on the latest EF Core 8/9 features, publishing courses on Pluralsight (her EF Core 8 Fundamentals course came out in early 2024). She also provides mentoring and consulting to teams adopting DDD with C#. Julie’s generous sharing of knowledge over the years and her continued active engagement make her an inspiration and top developer in the CSharp community.
- LinkedIn: Julie Lerman
- X (Twitter): @julielerman
- GitHub: julielerman
- Website/Blog: thedatafarm.com/blog
Wrap Up
These legends represent exceptional talent, making them extremely challenging to headhunt. However, there are thousands of other highly skilled IT professionals available to hire with our help. Contact us, and we will be happy to discuss your hiring needs.
Note: We’ve dedicated significant time and effort to creating and verifying this curated list of top talent. However, if you believe a correction or addition is needed, feel free to reach out. We’ll gladly review and update the page.